Reference project structure
The image below shows the project structure for which the script is designed. Most likely, it's different in your case, so adjust all paths to your needs.
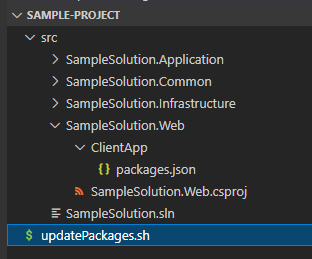
The updatePackages.sh
is in the root folder. The src
directory contains the solution file SampleSolution.sln
. All subfolders are related to particular projects and contain project files with the .csproj
extension. The web project SampleSolution.Web
handles SPA requests, and thus there is the ClientApp
directory containing the package.json
file.
Updating NuGet packages
The code below is responsible for updating all NuGet packages based on project files. It just:
- iterates over
.csproj
files and reads its content, - then iterates over each line of
.csproj
file, - if a line matches the regex (package definition) it takes a package name and updates it to the newest version.
(cd ./src && dotnet restore)
regex='PackageReference Include="([^"]*)" Version="([^"]*)"'
echo 'Updating nuget packages...'for csproj in ./src/*/*.csproj; do csprojFullPath=$(readlink -f "$csproj") while read -r line; do if [[ $line =~ $regex ]]; then package="${BASH_REMATCH[1]}" dotnet add "$csprojFullPath" package "$package" fi done <"$csproj"done
Updating NPM packages
To update all NPM packages I suggest using npm-check-updates package. To install it globally, run the command:
npm i -g npm-check-updates
This package is required to run the ncu -u
command in the script below. The script iterates over all package.json
files in the src
subdirectories (only one in the reference project) and uses the ncu
to bump the packages to the latest versions.
echo 'Updating npm packages...'for packageJson in ./src/*/package.json; do dirName=$(dirname "$packageJson") (cd "$dirName" && ncu -u --packageFile package.json && npm install)done
Usage in CI/CD
You can freely use these scripts in your CI/CD. Remember not to push the changes without a manual check! In my case "packages update" job is triggered once per month. This job:
- updates all packages,
- creates a branch and pushes all changes,
- creates a Jira task on my team's scrum board.
Then we manually verify if everything works fine.
Conclusion
Be always up to date, and then all updates go more smoothly. Remember that every package update may break your code, so spend some time performing smoke tests of your app. You can find the whole script on my Github.